We use cookies to make your experience better. To comply with the new e-Privacy directive, we need to ask for your consent to set the cookies. Learn more.
How to become an algorithmic trader - Lesson 2
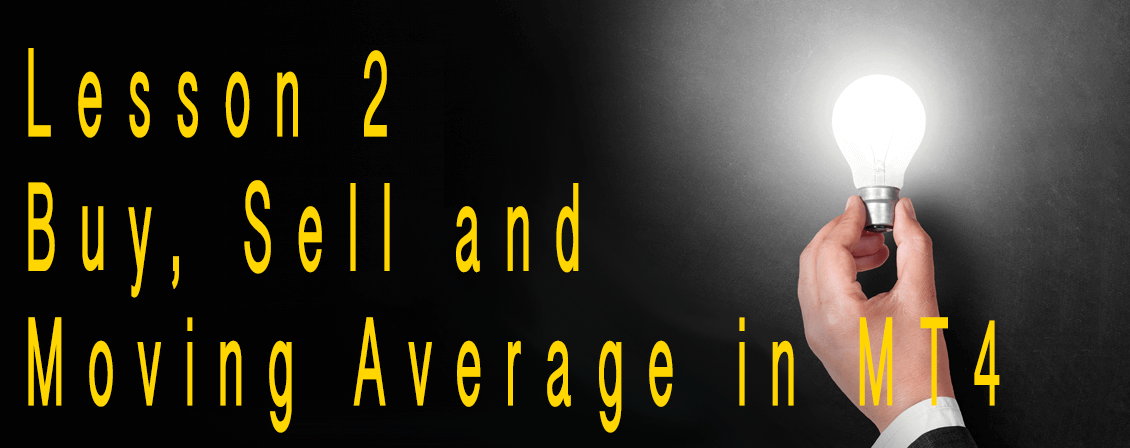
Become an Algorithmic Trader - Learn how to Buy, sell and use simple technical indicators
The simplest technical indicator: Moving Average
The aim of these lessons is to provide you with some simple hints to start coding an Expert Advisor. I will not explain you how to get coding skills or how to use the if statement. I will give you the instruments to understand how to open a position and how to read a technical indicator to do a trade. The easiest indicator that I would like to explain is Moving Average. How to tell an Expert Advisor to calculate a Moving Average?
One line of code is enough to have the Moving Average Indicator, as it follows:
double iMA(Symbol(),PERIOD_CURRENT,20,0,MODE_EMA,PRICE_OPEN,0);
the “double” before iMA() expression means that Metatrader expects to have a number with decimal positions as output for iMA() expression. All the stuff inside iMA brackets is the set of parameters needed to give a value to iMA.
- First parameter is the symbol, which means the Symbol name on the data of which the indicator will be calculated. NULL or Symbol() mean the current symbol
- Second parameter is the Timeframe. It can be any of ENUM_TIMEFRAMES enumeration values. PERIOD_CURRENT means the current chart timeframe. Values go from PERIOD_CURRENT to PERIOD_MN1. Read here for more detailed information about PERIOD
- Third parameter is the Averaging period for calculation that means how many candles it goes back to compute the average (in our example 20 candles)
- Fourth parameter is MA shift. Indicators line offset relate to the chart by timeframe
- Fifth parameter is Moving Average method. It can be any of ENUM_MA_METHOD enumeration values:
- MODE_SMA that calculates a simple moving average
- MODE_EMA that calculates an exponential moving average
- MODE_SMMA that calculates a smoothed moving average
- MODE_LWMA that calculates a Linear-weighted averaging
- Sixth parameter is Applied price. It means to which price compute the moving average. It can be any of the following:
- PRICE_CLOSE that means computed on Close price
- PRICE_OPEN that means computed on Open price
- PRICE_HIGH that means computed on the Maximim price for the period
- PRICE_LOW that means computed on the Minimum price for the period
- PRICE_MEDIAN that means computed on the Median price (High + low) / 2 for the period
- PRICE_TYPICAL that means computed on the Typical price (High + Low + Close) / 3 for the period
- PRICE_WEIGHTED that means computed on the Weighted price (High + Low + Close + Close) / 4
- Seventh parameter is the shift. Index of the value taken from the indicator buffer (shift relative to the current bar the given amount of periods ago).
Now we have an indicator to refer to in order to decide whether to put an order or not, we just miss the order function.
The OrderSend function in Metatrader 4
int OrderSend(Symbol(),OP_BUY,0.01,Ask,50,0,0,"My order",100,0,clrGreen);
This function is used to send an order and buy or sell a symbol from the Market.
Let’s now explain how it works.
- First parameter is the symbol, which means the Symbol name on the data of which the indicator will be calculated. NULL or Symbol() mean the current symbol
- Second parameter is the Operation type. It can be one of the following values:
- OP_BUY that is used to Buy the symbol
- OP_SELLthat is used to Sell the symbol
- OP_BUYLIMIT that is used to set a Buy limit pending order
- OP_SELLLIMIT that is used to set a Sell limit pending order
- OP_BUYSTOP that is used to set a Buy stop pending order
- OP_SELLSTOP that is used to set a Sell stop pending order
- Third parameter is the Number of lots you want to buy. It depends on the Broker and the leverage you are using. The lower the value, the smaller the amount of that symbol you buy
- Fourth parameter is the Order price. It is the price you want to refer to buy/sell. Usually it is used Ask price to buy and Bid price to sell
- Fifth parameter is the Maximum price slippage for buy or sell orders. It means that you accept to buy/sell with a margin. In this case we put 50 points of slippage that means we accept to buy until the Ask price goes up to Ask + 50 points
- Sixth and Seventh parameters are Stop loss and Take profit levels. We did not set them in this example, but they can be easily thought as Ask - spread and Ask + spread for buy orders and vice versa for sell in the open orders
- Eighth parameter is just a comment
- Ninth parameter is the Magic number. May be used as user defined identifier. For example if your server shuts down and you need to reset you Metatrader, all you connections between opened positions and Expert Advisors are lost without the Magic number. Magic number is a connection for your Expert Advisor to remember all the positions opened
- Tenth parameter is the order expiration time, buti s used just for pending orders (we will not explain this parameter here)
- Eleventh parameter is the Color of the opening arrow on the chart. If parameter is missing or has CLR_NONE value opening arrow is not drawn on the chart. This is useful for backtests in order to see clearly all the positions opened and closed with different colors directly from the chart
We have now explained two main functions that can help us building a first Expert Advisor. We will try to write a simple Expert Advisor together during the next lesson.